C# Barcode Generation Tutorial
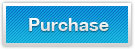
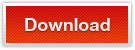
With our professional barcode generating control, you are able to create most popular linear & Matrix barcodes in C# for .NET framework applications. They are includes:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- Numeric Barcodes: Identcode, Leitcode, Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.
Features of Barcode Creating SDK for C#.NET
Barcode Creator SDK in C# .NET helps you generate most common linear and matrix barcodes that you probably need in your C# .NET applications. It is entirely built in .NET 2.0 and supports .NET 2.0, 3.0, 3.5, 4.0. Bar coding in C# .NET classes and console applications is easy. Using this Barcode Creator with C# .NET creating code, barcodes can also be easy to be generated and printed from ASP.NET web projects, Microsoft Windows Forms, SQL Server Reporting Services (SSRS), and Crystal Reports.
Barcode Images Generated by C#.NET Barcode Maker
It is able to export barcode as image formats in gif, jpeg, png, bmp, and tiff with C# code. Barcodes can be rotated to 0, 90, 180, or 270 degrees based on specific requirements. The image size and other properties may be adjusted to meet the users' specifications. High quality barcodes are supported by this product without any distortion.
Configuring Barcode Properties Using C#.NET
Full functional features of barcode creator in C# .NET make the operation of barcode easy and simple. Using this creator, the appearance of the human readable characters on linear barcodes can be enabled or disabled. The checksum character that encoded in linear barcodes can be displayed for human reading. Barcode Creator provided the functionality of configuring the colors of foreground and background in C#.NET. It is easy to encode the returns, tabs and other functions into your C# .NET applications. Bearer bar is also easy to be generated for linear barcodes including ITF14.
Add Barcode Control to C#.NET Template Projects
Creating Barcodes Using .NET Barcode Generator Windows Form Control
To create barcodes in C# Windows Forms applications, please follow the below procedures:
- Download the .NET barcode trail version package and unzip it to a folder
- Open your exist C# Window Application project or create a new C# project
- Add KeepDynamic.BarCode.Windows.dll to your C# project reference
- Add KeepDynamic.BarCode.Windows.dll to windows project toolbox
- Drag the one of the BarCodeControl, DataMatrixControl, PDF417Control, QRCodeControl item to your windows forms
Generating Barcodes in ASP.NET Web Applications using ASP.NET Barcode Control
To create barcodes in C# ASP.NET Web Forms applications, do the following procedures:
- Download the .NET barcode package and extract the files from it
- Open the C# ASP.NET Web application that you are going to adding barcodes or create a new web project in C# ASP.NET
- Add KeepDynamic.BarCode.AspNet.dll to your ASP.NET project reference
- Add KeepDynamic.BarCode.AspNet.dll to ASP.NET project toolbox
- Copy folder barcode from downloaded trial package to your ASP.NET project
- Drag the one of the BarCodeControl, DataMatrixControl, PDF417Control, QRCodeControl item to your ASP.NET Web form pages
How to Generate Linear (1D) Barcodes in C#.NET Class?
The following C# .NET code illustrates how to generate a linear barcode in C# class:
// Create linear barcode object in C#
BarCode code128 = new BarCode();
// Set barcode symbology type to Code-128 in C#
code128.SymbologyType = SymbologyType.Code128;
// Set barcode value in C#
code128.CodeText = "Code128";
// Adjust Code 128 barcode image size
code128.BarCodeWidth = 150;
code128.BarCodeHeight = 90;
// Set barcode drawing image format to PNG in C#
code128.Format = System.Drawing.Imaging.ImageFormat.Png;
// Generate barcode & encode to the png image in C#
code128.drawBarcode("C://barcode-code128-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = code128.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...
code128.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = code128.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
code128.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
code128.drawBarcode(stream);
How to Generate Data Matrix Barcodes in C#.NET Class?
The following C# .NET code illustrates how to generate a Data Matrix barcode in C# class:
// Create Data Matrix barcode object in C#
DataMatrix barcode = new DataMatrix();
// Set Data Matrix barcode value in C#
barcode.CodeText = "Data Matrix in C#";
// customize data matrix barcode image size settings
barcode.X = 4;
barcode.TopMargin = 4;
barcode.RightMargin = 4;
barcode.LeftMargin = 4;
barcode.BottomMargin = 4;
// Set Data Matrix barcode data mode
barcode.DataMode = DataMatrixDataMode.ASCII;
// Generate Data Matrix barcode & encode to the png image in C#
barcode.drawBarcode("C://barcode-datamatrix-csharp.png");
How to Generate PDF-417 Barcodes in C#.NET Class?
The following C# .NET code illustrates how to generate a PDF-417 barcode in C# class:
// Create PDF417 barcode object in C#
PDF417 barcode = new PDF417();
// Set PDF-417 barcode value in C#
barcode.CodeText = "PDF417 in C#";
// set unique PDF 417 barcode image settings
barcode.ColumnCount = 3;
barcode.RowCount = 10;
barcode.X = 3;
barcode.XtoYRatio = 0.3333333f;
// Set PDF-417 drawing image format to PNG in C#
barcode.Format = System.Drawing .Imaging .ImageFormat.Png;
// Generate PDF-417 barcode & encode to the png image in C#
barcode.drawBarcode("C://barcode-pdf417-csharp.png");
How to Generate QR-Code Barcodes in C#.NET Class?
The following C# .NET code illustrates how to generate a QR-Code barcode in C# class:
// Create QR-Code barcode object in C#
QRCode barcode = new QRCode();
// Set QR-Code barcode value in C#
barcode.CodeText = "QRCode in C#";
// Set QR Code module size
barcode.X = 4;
// Set QR Code quiet zone
barcode.BottomMargin = 16;
barcode.LeftMargin = 16;
barcode.RightMargin = 16;
barcode.TopMargin = 16;
//Set QR Code error correction level
barcode.ECL = QRCodeECL.L;
// Set QR-Code drawing image format to PNG in C#
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
// Generate QR-Code barcode & encode to the png image in C#
barcode.drawBarcode("C://barcode-qrcode-csharp.png");