C# .NET PDF-417 Barcode Generator Library
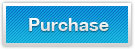
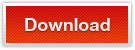
C# .NET PDF-417 Barcode Generator Library is a developer-library, which is used to create, generate, or encode pdf417 barcode for .NET framework apps in Visual Studio 2005/2008/2010 using C# class codes.
Overview - C# .NET PDF-417 Barcode Generator Library
2D barcode PDF417, also known as Portable Data File 417, PDF 417, PDF417 Truncated, is a stacked linear barcode symbol. Similar to other 2d barcode types, PDF417 barcode has high-density data capacity and strong error correction ablility. This C#.NET barcode generating library is designed to generate & create PDF417 barcode images using C# class codes.
Related solutions for generating PDF417 barcode image using C# class codes:
Features - C# .NET PDF-417 Barcode Generator Library
Technologies of PDF 417 Barcode C# .NET Creator Control
- C# .NET PDF 417 Barcode Creator makes PDF 417 barcodes in your C# .NET applications easily
- This product is entirely built in .NET 2.0 and supports the greater version, including .NET 2.0, 3.0, 3.5, 4.0
- this C# .NET barcoding control support generating PDF417 barcodes in C# .NET classes and Console applications, ASP.NET web service, Windows Forms Project
- Using this C#.NET PDF417 barcode generator dll, it is easy to generate PDF417 barcode in Reporting Services, RDLC Reports and Crystal Reports
Image Settings of Generated PDF417 Barcodes in C#.NET Application
Full functional features of C# .NET PDF-417 Barcode Creator make the generation of PDF-417 barcode easy and simple. For instance:
- Offer several image formats to generate & save PDF417 barcodes in C#.NET class, like gif, jpeg, png, bmp, and tiff
- Provide four creating orientations(0, 90, 180, or 270 degrees) to generate PDF417 barcode image using C# code
- Support PDF417 barcode size customization in C#.NET application
- Offer different data modes to encode data into PDF417 barcode using C# code
- Able to generate PDF417 barcode with different Error Correction Levels in C#.NET project
- Free to choose the rows and columns for PDF417 barcode generation in C#.NET class
Generate & Create PDF417 Barcodes using C#.NET Class Code
//Create a new PDF417 barcode object in C#.NET,
PDF417 barcode = new PDF417();
//Assign the data that are encoded into PDF417 barcode symbology.
barcode.CodeText = "Abc1234567";
/*
Choose DataMode for PDF417 barcode that is going to generate,
1. Text Compaction mode encodes values 9,10,13 & 32-127 of ASCII
2. Bytes Compatication mode encodes all 256 byte values of ASCII
3. Numeric Compatication mode encodes numeric digits 0 through 9
*/
barcode.DataMode = PDF417DataMode.Auto;
//Set the Error Correction Level(ECL) to PDF417
//Disenable the Truncated option to PDF417 symbology
barcode.ECL = PDF417ECL.ECL_4;
barcode.Truncated = false;
//Set the number of rows and columns to PDF417
barcode.RowCount = 9;
barcode.ColumnCount = 6;
//Enable the option of ProcessTilde when the tilde character "~" is necessary
barcode.ProcessTilde = true;
//Set the PDF417 barcode graphic measurements as Pixel
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Assign the values of bar module width to PDF417 barcode
barcode.X = 2;
// Customize PDF417 barcode symbol size
barcode.BarCodeWidth = 350;
barcode.BarCodeHeight = 70;
//Set the margins around the PDF417 barcode symbology
barcode.LeftMargin = 4;
barcode.RightMargin = 4;
barcode.TopMargin = 4;
barcode.BottomMargin = 4;
/*
Set the resolution of PDF417 barcode image that is drawn to,
and change the PDF417 barcode image orientation
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
/*
Set PDF417 barcode drawing image format to PNG in C#
Generate PDF417 barcode image in C#
*/
barcode.Format = System.Drawing .Imaging .ImageFormat.Png;
barcode.drawBarcode("C://barcode-pdf417-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
C#.NET PDF418 Barcode Generator Library Supported Barcode Types
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.