C# QR Code Barcode Generator
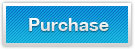
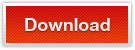
C# QR Code Barcode Generating Component encode & print QR Code in .NET projects using C# with Visual Studio.
Overview - C#.NET QR Code Generator
QR Code C# .NET Barcode Generator for the creation of QR Code, a two-dimensional code, consisting of black modules arranged in a square pattern on a white background, in your C# .NET framework projects. It is also known as Denso Barcode, QRCode, Quick Response Code, and compatible with the ISO/IEC18004.
Here are links to popular barcoding solutions for generating QR Code image in C#.NET
Features - C#.NET QR Code Encoding Library
Technologies of QR Code Barcode C# .NET Creating Control
- An easy-to-use QR Code barcode generating control with strong barcode generating functions for C#.NET class applications
- Completely developed in .NET 2.0, this C#.NET QR Code barcode generator library supports barcode generation in .NET 2.0, 3.0, 3.5, 4.0 framework
- Built in 100% managed C# code, this C#.NET barcoding library can generate QR Code images in ASP.NET web application, WinForms project and Console application
- Able to generate & create QR Code barcode images in SQL Server Reporting Services (SSRS), RDLC Local Reports and Crystal Reports using C# code
Generated QR Code Images Using C#.NET Barcoding Control
- Image formats supported by this QR Code C#.NET barcode Creator include gif, jpeg, png, bmp, and tiff
- QR Code Barcodes can be set to 0, 90, 180, or 270 degrees based on specific requirements in C#.NET project
- Strong QR Code image sizing feature are supported by this C#.NET barcode generator library
- Module size, QR Code symbol size, QR code versions and quiet zone width are free to customize using this C#.NET barcode creating control
- Offer ProcessTilde function to encode the returns, tabs and other functions into C# .NET QR Code barcode images
- Information such as text, URL or other data can be encoded into QR Code symbol and may be decoded by dedicated QR Code readers and camera phones
- Generated QR Code barcode images meet latest industry-standards
How to Create QR Code in .NET Applications Using C# Codes
After intalling KeepDynamic.BarCode.AspNet.dll or KeepDynamic.BarCode.Windows.dll to your .NET project, you can use follwing C# samlpe codes to generate high-quality QR Code image.
//Create a new QR Code barcode object in C#.NET,
QRCode barcode = new QRCode();
//Assign the data value to QR Code barcode symbology and QR Code encodes variable data length
barcode.CodeText = "Abc1234567";
/*
Choose DataMode for QR Code barcode that is going to generate
Numeric data mode encodes numbers 0 through 9;
AlphaNumeric encodes digits 0 to 9, all uppercase letters, and special characters(space, $ % * + - . / :);
byte data mode encodes characters define in ISO/IEC 8859-1;
Kanji data mode encodes Kanji characters;
*/
barcode.DataMode = QRCodeDataMode.Auto;
//Select a QR Code version from QR Code version list
//Set the Error Correction Level(ECL) to QR Code
barcode.Version = QRCodeVersion.V6;
barcode.ECL = QRCodeECL.L;
//Enable the option of ProcessTilde when the tilde character "~" is necessary
barcode.ProcessTilde = true;
//Set the QR Code barcode graphic measurements as Pixel
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Assign the values of bar module width to QR Code barcode
barcode.X = 4;
//Set the margins around the QR Code barcode symbology
barcode.LeftMargin = 16;
barcode.RightMargin = 16;
barcode.TopMargin = 16;
barcode.BottomMargin = 16;
/*
Set the resolution of QR Code barcode image that is drawn to,
and change the QR Code barcode image orientation
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
/*
Set QR Code barcode drawing image format to PNG in C#
Generate QR Code barcode image in C#
*/
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
barcode.drawBarcode("C://barcode-qrcode-csharp1.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Generate 1D & 2D Barcodes using C# QR Code Generator SDK
The
C#.NET Barcode Generator can create most common linear (1d) and matrix (2d) bar code standards in C#.NET projects, including:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.