C# Code 39 Barcode Creator / Generator Control
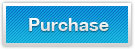
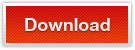
C# Code 39 Barcode Creator / Generator Control supports Code 39 barcode generation in C# .NET class application.
Overview - Code 39 Barcode Generator Library for C# .NET Application
Keepdynamic.com provides Code-39 C# .NET Barcode Generator Library for the creation/generation of Code 39 barcodes in your C# .NET framework projects. Code 39 is an alphanumeric, discrete, and variable-length barcode symbology. In code 39 barcode image symbol, a fixed pattern of bars represents a character. Each character is made up of 9 bars (including 3 wider bars). Thereby this barcode is also known as Code 3 from 9, USS Code 39, Code 3/9, Code 3 of 9.
Related barcoding solutions for generating & creating Code 39 barcode image using C#.NET class codes:
Features - C# .NET Code 39 Barcode Generator Library
Technologies of Code 39 C# .NET Barcode Generating Library
- C# .NET Code-39 Barcode Creator is used to create & generate Code 39 barcodes in your C# .NET applications
- Code 39 supports 43 characters and an additional character (which is the '*', used as a start/stop character)
- This Code 39 barcoding library for C# .NET Application is entirely built in .NET 2.0 and supports .NET 2.0, 3.0, 3.5, 4.0 & above
- It can generate Code-39 barcode images in C# .NET classes and console applications
- this C# .NET Barcode Creator Library can also generate Code 39 barcode images in ASP.NET web projects, Microsoft Windows Forms
- This C# Code 39 barcode generaring library also support Code 39 barcode generation in RDLC Local Reports, RDLC Local Report and Crystal Reports
- Generated Code 39 barcode images by this C#.NET barcode generator are compatible with most printers
Barcode Image Settings of Code 39 C# .NET Barcode Generating Library
- Generate & save Code 39 barcode images to gif, jpeg, png, bmp, and tiff image files in C#.NET application
- Rotate created Code 39 barcode images to 0, 90, 180, or 270 degrees using C# code
- Adjust generated Code 39 barcode images size-related properties, like bar width, barcode symbol size and wide-to-narrow ratio using C#
- Compute & add checksum characters to generated Code 39 barcode images in C#.NET class
- Able to display to hide human-readable text of Code 39 barcode images in C#.NET class application
Free C# Code for Code 39 Barcode Generation in .NET Application
/*
Create a new linear barcode object in C#.NET,
and change the barcode symbology type to Code 39
*/
BarCode barcode = new BarCode();
barcode.SymbologyType = SymbologyType.Code39;
/*
Assign characters that are encoded into Code 39 barcode symbology.
Encodable character set of Code 39 is as follow,
full alphanumeric A to Z and 0 to 9
special characters: space / $ % . + -
start/stop character: *
*/
barcode.CodeText = "ABC0123";
//Enable checksum for Code 3 of 9 so that the check digit will be calculated automatically
barcode.EnableChecksum = true;
//Set the barcode graphic measurements as Pixel for Code 39
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Set the values of bar width and bar height for Code 39
barcode.X = 1;
barcode.Y = 60;
//Set the margins around the Code 39 symbol
barcode.LeftMargin = 10;
barcode.RightMargin = 10;
barcode.TopMargin = 10;
barcode.BottomMargin = 10;
// Set generated Code 39 barcode image size
barcode.BarCodeWidth = 200;
barcode.BarCodeHeight = 100;
/*
Set the resolution of Code 3of9 image that is drawn to,
and change the orientation of Code39 barcode image
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
//Set the wide-to-narrow ratio for Code 39
barcode.WideNarrowRatio = 3.0f;
/*
Display the characters encoded into the Code 39 symbology
Add start and stop characters to Code 3of9 barcode image
Set the font style of the characters encoded into Code 39 barcode
*/
barcode.DisplayCodeText = true;
barcode.DisplayStartStopChar = true;
barcode.CodeTextFont = new System.Drawing.Font("Arial", 11f, System.Drawing.FontStyle.Regular);
/*
Set Code 39 barcode drawing image format to PNG in C#
Generate Code 39 image in C#
*/
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
barcode.drawBarcode("C://barcode-code39-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Generate 1D & 2D Barcodes Using Code 39 C# .NET Barcode Generating Library
Code 39 C# .NET Barcode Generating Library cannot only create Code 39 barcode images in C# .NET application, but generate other 1d and 2d barcode images in C# .NET class projects.
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.