Code 39 ASP.NET Barcode Generator Library
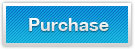
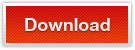
Code 39 ASP.NET Barcode Generating Class Library is used to insert, create, or design Code 39 barcodes in ASP.NET web server applications using C# and VB.NET.
Code 39 ASP.NET Barcode Generator Library - Overview
Code-39 ASP.NET Barcode generator is a fully-functional linear barcode creator component for ASP.NET web applications. Using this ASP.NET Code 39 barcode generating control, users can create Code 39 barcode image (also called USS Code 39, Code 3/9, Code 3 of 9, USD-3, Alpha39, or Type 39), in your ASP.NET web server applications.
Other widely-used barcoding solutions for generating & printing Code 39 barcode image in .NET projects:
Features - Code 39 ASP.NET Barcode Generator Component
ASP.NET Code 39 Barcode Generator Control- Technology
- Code 39 Barcode Generator for ASP.NET is entirely written in managed C#.NET
- ASP.NET Code 39 barcoding library is compatible with .NET framework 2.0, 3.0, 3.5, 4.0 and greater
- The ASP.NET Code 39 barcode creator may be easily integrated and redistributed into your ASP.NET web server projects without any license keys or activation
- It supports all web browsers for Code 3 of 9 barcode images generation on the server side
- Using this ASP.NET Code 39 barcoding library, users can generate & create Code 39 barcode images in ASP.NET web services, C#.NET applications, VB.NET class project & Console applications
ASP.NET Code 39 Barcode Generator Control - Image Settings
- Generated Code 39 barcode images can be saved to JPEG, GIF, TIFF, BMP, and PNG image format files in ASP.NET applications.
- Free to design the size-related properties of created Code 39 barcode images in ASP.NET, like bar width, wide to narrow ratio and barcode symbol size.
- Four generating directions(0, 90, 180, or 270 degrees) are available for Code 39 barcode image generation.
- Printing resolutions for created Code 39 barcode images are user-defined.
- Able to display or hide human-readable text of generated Code 39 barcode images in ASP.NET website
- Checksum character can be automatically added to Code 39 barcode images in ASP.NET applications
Generation Guidance - Code 39 ASP.NET Barcode Generator Component
This ASP.NET Code 39 barcoding library offers users three ways to generate & create Code 39 barcode images.
How to Create Code 39 Barcode in ASP.NET Web Controller
- Download & unzip the KeepDynamic.BarCode.AspNet.Eval
- Add KeepDynamic.BarCode.AspNet.dll from the file Library to your ASP.NET project and Visual Studio Toolbox
- Copy "linear.aspx", "linear.aspx.cs" from barcode folder in Documentation to your ASP.NET project
- Drag & drop BarCodeControl from the Toolbox to your ASP.NET project and a barcode image will be generated
- Click generated barcode image, change the value of SymbologyType to Code39 and adjust the value of CodeText to Code 39 barcode encodable characters(numbers 1-9 & upper-class alphanumeric characters A-Z) in the Properties window
- Run the ASP.NET project and a standard Code 39 barcode image will be created
How to Generate Code 39 Barcode in ASP.NET Class Applications
- Integrate KeepDynamic.BarCode.AspNet.dll from the file Library to your ASP.NET project by adding reference
- Copy following C# or VB.NET class code to generate Code 39 barcode images in ASP.NET web applications.
Generate Code 39 barcode images in ASP.NET using C#
// generate linear barcode object
BarCode barcode = new BarCode();
// Set barcode type to Code-39
barcode.SymbologyType = SymbologyType.Code39;
// Enter encodable value to Code39 barcode
barcode.CodeText = "CODE39";
//Display Code 39 start / stop character
barcode.DisplayStartStopChar = true;
// Add checksum character to Code 39
barcode.EnableChecksum = true;
// set wide & narrow bar width ratio
barcode.WideNarrowRatio = 3;
// set Code 39 barcode image settings
// Set bar width of Code 39
barcode.X = 3;
// set Code 39 barcode image
barcode.BarCodeWidth = 500;
barcode.BarCodeHeight = 90;
// Set Code39 drawing image format to PNG
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
// save generated barcode images to png image file using C#
barcode.drawBarcode("C://kdcode39.png");
Generate Code 39 barcode images in ASP.NET using VB.NET
' generate linear barcode object
Dim barcode As New BarCode()
' Set barcode type to Code-39
barcode.SymbologyType = SymbologyType.Code39
' Enter encodable value to Code39 barcode
barcode.CodeText = "CODE39"
'Display Code 39 start / stop character
barcode.DisplayStartStopChar = True
' Add checksum character to Code 39
barcode.EnableChecksum = True
' set wide & narrow bar width ratio
barcode.WideNarrowRatio = 3
' set Code 39 barcode image settings
' Set bar width of Code 39
barcode.X = 3
' set Code 39 barcode image
barcode.BarCodeWidth = 500
barcode.BarCodeHeight = 90
' Set Code39 drawing image format to PNG
barcode.Format = System.Drawing.Imaging.ImageFormat.Png
' save generated barcode images to png image file using VB.NET
barcode.drawBarcode("C://kdcode39.png")
How to Generate Code 39 Barcode in IIS using ASP.NET Barcoding Library
- Find folder barcode in the folder Documentation of downloaded trial package.
- Copy cotent of folder barcode to your IIS project, eg:C:\interpub
- Create a new virtual directory in your IIS named "barcode", and connect it to the above barcode folder in the C:\interpub
- Restart your Microsoft IIS and navigate to:
http://YourDomain:Port/barcode/linear.aspx?symbology-type=4&code-text=39393939&x=1&left-margin=10&right-margin=10 - Now, a Code 39 barcode is created on a page. Besides, you can use the following image tag to generate a Code 39 barcode on your html or aspx pages.
<img src="http://YourDomain:Port/barcode/linear.aspx?symbology-type=4&code-text=39393939&x=1&left-margin=10&right-margin=10" />
Note: X refers to bar width and code-text refers to encodable characters.
Barcode Types - Code 39 ASP.NET Barcode Generator Component
- 2D Barcodes: QR Code in ASP.NET, PDF417 in ASP.NET and Data Matrix in ASP.NET.
- Alphanumeric Barcodes: Code 39 in ASP.NET, Code 93 in ASP.NET, Code 128 in ASP.NET and GS1-128 (UCC/EAN-128) in ASP.NET.
- UPC / EAN Barcodes: EAN 13 in ASP.NET, EAN 8 in ASP.NET, UPC A in ASP.NET, UPC E in ASP.NET, ISBN in ASP.NET and ISSN in ASP.NET.
- Numeric Barcodes: Code 11 in ASP.NET, Codabar in ASP.NET and MSI Plessey in ASP.NET.
- Code 2 of 5 based Barcodes: Standard 2 of 5 in ASP.NET, Interleaved 2 of 5 in ASP.NET and ITF14 in ASP.NET.
- Postal Barcodes: Intelligent Mail Barcode in ASP.NET (USPS OneCode Solution), USPS POSTNET in ASP.NET, USPS PLANET in ASP.NET, Identcode in ASP.NET, Leitcode in ASP.NET and RM4SCC in ASP.NET.