.NET Barcode Generation Tutorial
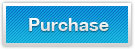
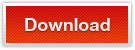
How to Generate Linear & 2D Barcodes in .NET Projects?
.NET Barcode Generator offers the most affordable .NET barcode generator for barcode .NET professionals.
Creating Barcodes using .NET Barcode Generator Windows Form Control
To create barcodes in C# & VB.NET Windows Forms applications, do the following procedures:
- Add KeepDynamic.BarCode.Windows.dll to your C#, VB.NET project reference
- Add KeepDynamic.BarCode.Windows.dll to windows project toolbox
- Drag the one of the BarCodeControl, DataMatrixControl,PDF417Control, QRCodeControl item to your windows forms
Creating Barcodes using ASP.NET Barcode Generator ASP.NET Web Form Control
To create barcodes in ASP.NET Web Forms applications, do the following procedures:
- Add KeepDynamic.BarCode.AspNet.dll to your C#, VB.NET project reference
- Add KeepDynamic.BarCode.AspNet.dll to windows project toolbox
- Copy folder barcode from downloaded trial package to your ASP.NET project
- Drag the one of the BarCodeControl, DataMatrixControl, PDF417Control, QRCodeControl item to your ASP.NET Web form pages
How to Generate Barcodes in C#.NET Class?
The following C# .NET code illustrates how to generate a barcode in C# class:
// Create linear barcode object in C#
BarCode code128 = new BarCode();
// Set barcode symbology type to Code-128 in C#
code128.SymbologyType = SymbologyType.Code128;
// Set barcode value in C#
code128.CodeText = "Code128";
// Adjust Code 128 barcode image size
code128.BarCodeWidth = 150;
code128.BarCodeHeight = 90;
// Set barcode drawing image format to PNG in C#
code128.Format = System.Drawing.Imaging.ImageFormat.Png;
// Generate barcode & encode to the png image in C#
code128.drawBarcode("C://barcode-code128-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = code128.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...
code128.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = code128.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
code128.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
code128.drawBarcode(stream);
How to Generate Barcodes in VB.NET Class?
The following VB.NET code illustrates how to generate barcodes in VB.NET class:
' Create linear barcode object in C#
Dim code128 As New BarCode()
' Set barcode symbology type to Code-128 in C#
code128.SymbologyType = SymbologyType.Code128
' Set barcode value in C#
code128.CodeText = "Code128"
' Adjust Code 128 barcode image size
code128.BarCodeWidth = 150
code128.BarCodeHeight = 90
' Set barcode drawing image format to PNG in C#
code128.Format = System.Drawing.Imaging.ImageFormat.Png
' Generate barcode & encode to the png image in C#
code128.drawBarcode("C://barcode-code128-vb.net.png")
' other barcode outputting ways:
' to byte array
byte[] barcodeInBytes = code128.drawBarcodeAsBytes()
' output generated barcode to Graphics object
Graphics graphics = ...
code128.drawBarcode(graphics)
' output generated barcode to Bitmap object
Bitmap barcodeInBitmap = code128.drawBarcode()
' draw created barcode to HttpResponse object
HttpResponse response = ...
code128.drawBarcode(response)
' save generated barcode images to Stream object
Stream stream = ...
code128.drawBarcode(stream)
.NET Barcode Generator Supported Barcode Types
.NET Barcode Generator supports most common linear (1d) and matrix (2d) bar code standards, including:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- Numeric Barcodes: Identcode, Leitcode, Codabar, Code-11, RM4SCC and MSI Plessey.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5, ITF-14.
- Postal Barcodes:POSTNET, PLANET and USPS Intelligent Mail Barcode (OneCode).