ASP.NET Barcode Generator Control Tutorial
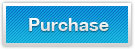
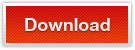
ASP.NET Barcode Generator offers the most affordable .NET barcode generator licenses for integrating barcodes into ASP.NET web projects. You will learn how to generate linear and 2D barcodes in ASP.NET web applications by using our ASP.NET server barcode control from this guide. ASP.NET barcode generator is an easy-to-use barcode control for ASP.NET Web solutions. After installing this control into your ASP.NET project, you can easily create quality 1D/2D barcode images in your ASP.NET web site / ASP.NET web applications. This barcode component supports generating linear and 2d barcodes with flexible size and dynamic resolution. And it will surely help you get desired barcode images for displaying and printing in a simple way.
1D/linear and 2D/matrix barcode symbologies that can be created by the ASP.NET barcode generator control include:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode, Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.
How to Create Barcodes for ASP.NET Web Template Projects
This tutorial gives a detailed illustration of how to instal the ASP.NET barcode generator control in Microsoft Visual Studio, and how to generate linear barcode symbology and two dimensional barcodes for ASP.NET application. To create linear barcode Code 39 in ASP.NET Web Forms applications, please do the following procedures:
- Download the ASP.NET barcode generator from the right top Download link and unzip the ASP.NET barcode package
- Open your Microsoft Visual Studio, and Create an ASP.NET Web application
- Copy the KeepDynamic.BarCode.AspNet.dll file to the C# or VB.NET project directory of the web application
- Add a reference to KeepDynamic.BarCode.AspNet.dll assembly in your ASP.NET project. The ASP.NET barcode generator is going to be used for a component library.
- After the barcode controls displayed in your Toolbox, you can create barcode image in your ASP.NET Web project
- Copy "linear.aspx", "linear.aspx.cs" from the barcode folder to your asp.net project
- Drag and drop BarCodeControl to your Web form pages in ASP.NET design view
- Change symbology type to Code 39 in the Properties window.
- Run the asp.net project and you will see a Code 39 barcode image
How to Print Barcodes in IIS using ASP.NET Barcode Generator
Apart from generating barcode images in Visual Studio developing platform, you can also generate barcode images in IIS using our ASP.NET barcoding component. Following are detailed steps to generate linear barcode Code 39 image:
- Find folder barcode in the downloaded trial package.
- Copy cotent of folder barcode to your IIS project, eg:C:\demo
- Create a new virtual directory in your IIS named "barcode", and connect it to the above barcode folder in the C:\demo
- Restart your Microsoft IIS and navigate to:
http://YourDomain:Port/barcode/linear.aspx?symbology-type=4&code-text=39393939&x=1&left-margin=10&right-margin=10
- Now, a Code 39 barcode is created on a page. Besides, you can use the following image tag to genrate a Code 39 barcode on your html or aspx pages:
<img src="http://YourDomain:Port/barcode/linear.aspx?symbology-type=4&code-text=39393939&x=1&left-margin=10&right-margin=10" />
Note: X refers to bar size, code-text refers to encodable characters.
How to Generate Barcodes in ASP.NET Using C# Class?
After you integrate our ASP.NET barcode generating dll KeepDynamic.BarCode.AspNet.dll into your ASP.NET web project by adding reference, you can generate & print linear and 2d barcode images using C# class codes.
// generate linear barcode object in C#
BarCode barcode = new BarCode();
// Set barcode type to Code-39 in C#
barcode.SymbologyType = SymbologyType.Code39;
// Enter encodable value to Code39 barcode in C#
barcode.CodeText = "CODE39";
// Set Code39 drawing image format to PNG in C#
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
// save generated barcode images to png image file using C#
barcode.drawBarcode("C://barcode-code39-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
How to Create Barcodes in ASP.NET Applications Using VB.NET?
Before you copy & paste following VB.NET clas code, please make sure you have installed KeepDynamic.BarCode.AspNet.dll to your ASP.NET applications.
' Create linear barcode object in VB.NET
Dim barcode As New BarCode()
' Set barcode symbology type to Code-39 in VB.NET
barcode.SymbologyType = SymbologyType.Code39
' Set Code39 barcode value in VB.NET
barcode.CodeText = "CODE39"
' Set Code39 drawing image format to PNG in VB.NET
barcode.Format = System.Drawing.Imaging.ImageFormat.Png
' Generate Code-39 barcode & encode to the png image in VB.NET
barcode.drawBarcode("C://barcode-code39-csharp.png")
pre class="csharpcode">' other barcode outputting ways:
' to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes()
' output generated barcode to Graphics object
Graphics graphics = ...
barcode.drawBarcode(graphics)
' output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode()
' draw created barcode to HttpResponse object
HttpResponse response = ...
barcode.drawBarcode(response)
' save generated barcode images to Stream object
Stream stream = ...
barcode.drawBarcode(stream)
ASP.NET Barcode Generator Technologies
This ASP.NET Barcode Creator is totally written in managed C# with strong name signatures and signed DLLs. It is compatible with .NET 2005 and greater developments and supports all web browsers displaying GIF, JPEG or PNG images on any operating system. ASP.NET Barcode Creator may make 1D and 2D barcodes in .NET Classes and console applications.
Barcode Images Generated by ASP.NET Barcode Generator
This ASP.NET Barcode Generator exports created barcode to multiple image formats such as: GIF, JPEG, PNG, BMP, TIFF, and WMF. The settings of ASP.NET barcode images are all controllable, such as the color of barcode background and bar, barcode image quality, image rotation angle, the x-dimension, captions, customer defined resolution and etc.
Customization of ASP.NET Linear and Matrix Barcodes
This ASP .NET Barcode Generator provides multiple options to set barcode images. For instance, using text option, developers can enable or disable the human-readable characters in the linear barcode. Options of graphic configuration help users set the colors of background and bars. Image orientation may rotate generated barcode images to 0, 90, 180 or 270 degrees.