Java Barcode QR Code Generator
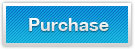
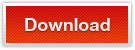
Java QR Code Barcode Generator offers the most affordable Java barcode generator for barcode Java professionals. It can generate & print QR Code in Java Class, Jasper Reports, iReport, BIRT.
Java QR-Code Encoder Introduction
QR-Code is also known as Denso Barcode, QRCode, Quick Response Code, JIS X 0510, ISO/IEC18004.
QR Code is a kind of 2-D (two-dimensional) symbology developed by Denso Wave (a division of Denso Corporation at the time) and released in 1994 with the primary aim of being a symbol that is easily interpreted by scanner equipment. QR Code is capable of handling all types of data, such as numeric and alphabetic characters, Kanji, Kana, Hiragana, symbols, binary, and control codes.
The symbol versions of QR Code range from Version 1 to Version 40. Each version has a different module configuration or number of modules (the module refers to the black and white dots that make up QR Code). "Module configuration" refers to the number of modules contained in a symbol, commencing with Version 1 (21 x 21 modules) up to Version 40 (177 x 177 modules). Each higher version number comprises 4 additional modules per side. Each QR Code symbol version has the maximum data capacity according to the amount of data, character type and error correction level. In other words, as the amount of data increases, more modules are required to comprise QR Code, resulting in larger QR Code symbols.
QR Code has error correction capability to restore data if the code is dirty or damaged. Four error correction levels are available for users to choose according to the operating environment.Raising this level improves error correction capability but also increases the amount of data QR Code size. To select error correction level, various factors such as the operating environment and QR Code size need to be considered. Level Q or H may be selected for factory environment where QR Code gets dirty, whereas Level L may be selected for clean environment with the large amount of data. Typically, Level M (15%) is most frequently selected. The QR Code error correction feature is implemented by adding a Reed-Solomon Code to the original data.
Java Barcode QR Code Generator - How to generate Barcode QR-Code in Java Class?
The following Java code illustrates how to generate a barcode in Java class:
QRCode barcode = new QRCode();
// set barcode properties
barcode.setCodeText("QRCode");
// draw and generate barcode to buffered image object
BufferedImage qrcodeImage = barcode.drawBarCode2Image();
Java Barcode QR Code Generator - How to Generate QR-Code Barcodes to Java Servlet Applicatons?
The following Java code illustrates how to generate a barcode in Java Servlet class:
public class BarcodeServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException
{
try {
QRCode barcode = new QRCode();
// set barcode properties
barcode.setCodeText("QRCode");
// draw and generate barcode to response's ServletOutputStream
ServletOutputStream servletoutputstream = response.getOutputStream();
barcode.drawBarCode2Stream(servletoutputstream);
} catch (Exception e) {
throw new ServletException(e);
}
}
}
Java Barcode QR Code Generator - How to Create QR-Code Barcodes through HTTP Request?
- Install Java Barcode Generator Servlet application to Tomcat or JBoss, developers need copy the barcode folder to your Tomcat application folder, and restart the Tomcat.
- After Tomcat restarted, open your browser and navigate to:
http://your_tomcat_url:port/barcode/qrcode?code-text=QRCODE - You can also insert barcodes in HTML or JSP pages.
<img src="http://your_tomcat_url:port/barcode/qrcode?code-text=QRCODE">
Java Barcode QR Code Generator - How to Draw & Print Barcode QR-Code to java.awt.Image Object?
The following Java source code shows how to generate a barcode in a java.awt.Image object:
public class BarcodeServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException
{
try {
QRCode barcode = new QRCode();
// set barcode properties
barcode.setCodeText("QRCode");
// draw and generate barcode to response's ServletOutputStream
ServletOutputStream servletoutputstream = response.getOutputStream();
barcode.drawBarCode2Stream(servletoutputstream);
} catch (Exception e) {
throw new ServletException(e);
}
}
Java QR-Code Properties
Class Properties |
HTTP Parameters |
Default |
Comments |
data |
data |
"" |
Barcode value to encode |
uom |
uom |
PIXEL |
Unit of meature for all size related settings. 0: pixel; 1: cm; 2: inch. Default is pixel |
moduleSize |
module-size |
1 |
Width of barcode module (narrow bar), default is 1 pixel |
leftMargin |
left-margin |
0 |
Barcode image left margin |
rightMargin |
right-margin |
0 |
Barcode image right margin |
topMargin |
top-margin |
0 |
Barcode image top margin |
bottomMargin |
bottom-margin |
0 |
Barcode image bottom margin |
resolution |
resolution |
72 |
Barcode image resolution in dpi |
rotate |
rotate |
0 |
Barcode rotate angle, valid values: 0, 90, 180, 270 |
processTilde |
process-tilde |
false |
Set the processTilde property to true, if you want use the tilde character "~" to specify special characters in the input data. Default is false.
~NNN: is used to represent the ASCII character with the value of NNN. NNN is from 000 - 255. |
dataMode |
data-mode |
0 (MODE_AUTO) |
- QRCode.MODE_AUTO: It allows encoding all 256 possible 8-bit byte values. This includes all ASCII characters value from 0 to 127 inclusive and provides for international character set support
- QRCode.MODE_ALPHANUMERIC: It allows encoding alphanumeric data (digits 0 - 9; upper case letters A -Z; nine other characters: space, $ % * + - . / : ).
- QRCode.MODE_BYTE: It allows encoding byte data (default: ISO/IEC 8859-1).
- QRCode.MODE_NUMERIC: It allows encoding numeric data (digits 0 - 9).
- QRCode.MODE_KANJI: It allows encoding Kanji characters.
|
ecl |
ecl |
0 (ECL_L) |
QR-Code Error Correction Level. Values:
QRCode.ECL_L (0),
QRCode.ECL_M (1),
QRCode.ECL_Q (2),
QRCode.ECL_H (3) |
eci |
eci |
3 |
|
fnc1Mode |
fnc1-mode |
0 (none) |
|
applicationIndicator |
ai |
0 |
|
isStructuredAppend |
structured-append |
false |
If true, then Structured Append is enabled. |
symbolCount |
symbol-count |
1 |
Set the number of total symbols which make the sequence. |
symbolIndex |
symbol-index |
1 |
Set the position of current symbol in the secuence (Start with 0). |
parity |
parity |
0 |
|
version |
version |
1 |
Set the position of current symbol in the secuence (Start with 0). |
Other Supported Barcode Types
Java Barcode Generator supports most common linear (1d) and matrix (2d) bar code standards, including:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Codabar, Code-11 and MSI Plessey.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: Identcode, Leitcode, POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode) and RM4SCC.