C# .NET Code 128 Barcode Generator Library
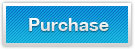
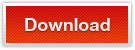
C# .NET Code 128 Barcode Generator Library is an advanced Code 128 barcoding control, designed to encode, embed & generate code128, code128a, code128b, and code128c using C# code in Visual Studio project templates.
Overview - C# .NET Code 128 Barcode Generator Control
Keepdynamic.com offers Code 128 C# .NET Barcode Generator for the generation of Code 128 barcodes, an alphanumeric barcodes with high-density data encoding capacity, in your C# .NET framework projects. Code 128 barcode symbology is also known as USS Code 128, Uniform Symbology Specification Code 128, Code 128A, Code 128B, Code 128C.
Related barcoding solutions for generating Code 128 image in C#.NET:
Features - C# .NET Code 128 Barcode Generator Control
Technologies of C# .NET Code 128 Barcode Generator Control
- C# .NET Code 128 Barcode Creator helps you generate Code 128 barcodes in your C# .NET applications
- All 128 characters of ASCII can be encoded into this Code 128 barcode symbol and created Code 128 barcode images are compatible with ISO/IEC 8859-1
- This Code 128 barcoding sdk is entirely built in .NET 2.0 and supports .NET 2.0, 3.0, 3.5, 4.0 & greater
- Using this C# .NET barcode generating sdk, users can generate Code 128 barcode images in C# .NET classes and console applications quite easily
- This C# .NET Barcode Generator Control can also generate & create Code 128 barcodes in ASP.NET web projects, Microsoft Windows Forms
- Using C#.NET barcoding dll, it is also quick to generate Code 128 barcode in SQL Server Reporting Services (SSRS), RDLC Local Reports and Crystal Reports
Barcode Image Customization of C# .NET Code 128 Barcode Generator Control
- Image formats supported by this Code 128 C#.NET barcode Creator include gif, jpeg, png, bmp, and tiff
- Generated Code 128 barcode images can be rotated to 0, 90, 180, or 270 degrees based on specific requirements in C#.NET applications
- The image size and other properties of Code 128 may be adjusted to meet the users' specifications in C#.NET applications
- Created Code 128 barcode images can have or have not human-readable text in C#.NET applications
- Free to set printing resolutions of generated Code 128 barcod images using C# code
Sample C# Code for Code 128 Barcode Image Generation in C# .NET Application
//Create a new linear barcode object in C#.NET
//Select the barcode type as Code 128 symbology from the barcode type list
BarCode barcode = new BarCode();
barcode.SymbologyType = SymbologyType.Code128;
/*
Set the data characters that are encoded into Code 128 barcode symbology.
Encodable data set of Code 128 contains,
All 128 ASCII characters (characters 0 to 127 inclusive).
Code 128 symbol encodes variable length of data characters
*/
barcode.CodeText = "Abc123";
/*
Enbable the checksum option for Code 128
so that the barcode generator computes check digit to Code 128 automatically
*/
barcode.EnableChecksum = true;
//Set the barcode graphic measurements as Pixel for Code 128
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Assign values of bar width and bar height to Code 128 barcode
barcode.X = 1;
barcode.Y = 80;
// Set generated Code 128 barcode image size
barcode.BarCodeWidth = 150;
barcode.BarCodeHeight = 120;
//Set the margins around the Code 128 symbol
barcode.LeftMargin = 10;
barcode.RightMargin = 10;
barcode.TopMargin = 10;
barcode.BottomMargin = 10;
//Assign a value to resolution for Code 128 image that is drawn to,
//Do not change the orientation of Code 128 barcode image
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
//Display the characters encoded into the Code 128 symbology and
//Set the font style of the characters encoded into Code 128
barcode.DisplayCodeText = true;
barcode.CodeTextFont = new System.Drawing.Font("Arial", 11f, System.Drawing.FontStyle.Bold);
//Set Code 128 barcode drawing image format to PNG in C# and
//Generate Code 128 image in C#
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
barcode.drawBarcode("C://code128-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Supported Barcode Types - C# .NET Code 128 Barcode Generator Control
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.