C# UPC-A Barcode Generator Library
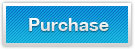
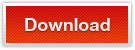
C# UPC-A Barcode Generator Library integrates UPC-A barcode generating feature into C#.NET applications. It offers free sample C# code to generate & create UPC-A barcode images in .NET project, ASP.NET web applications and Windows Forms project.
Overview - C# UPC-A Barcode Generator Library
Keepdynamic.com supply UPC-A C# .NET Barcode Generator for the generation of linear UPC-A barcodes in your C# .NET framework projects. UPC-A is also known as Universal Product Code version A, UPC-A Supplement 5/Five-digit Add-On, UPC-A Supplement 2/Two-digit Add-On.
Related barcode creating solutions for generating UPC-A barcode in .NET framework applications.
Features for C#.NET UPC-A Barcode Generator Library
Technologies of UPC-A C# .NET Creator Library
- Mature UPC-A barcode generating technologies for C#.NET project since 2004
- Built in .NET 2.0, this C#.NET barcoding control supports generating UPC-A barcodes in .NET 2.0, 3.0, 3.5, 4.0
- Generate & Create UPC-A barcode in C# .NET classes and Console applications
- Create & print UPC-A barcode in ASP.NET web projects, Microsoft Windows Forms, SQL Server Reporting Services (SSRS), RDLC Reports and Crystal Reports
Customization of C# .NET UPC-A Barcodes Generation
- Generate & draw UPC-A barcode images to gif, jpeg, png, bmp, and tiff files in C#.NET class
- Create UPC-A barcode images in 0, 90, 180, or 270 degrees based on specific requirements
- Generate & adjust UPC-A barcode images size and printing resolutions to meet users' specifications
- Generate & outout UPC-A barcode images with or without human-readable text using C# class code
Generate UPC-A Barcode using Free C#.NET Class Code in Visual Studio
/*
Create a new linear barcode object in C#.NET
and select the barcode symbology as UPC-A from the barcode type list
*/
BarCode barcode = new BarCode();
barcode.SymbologyType = SymbologyType.UPCA;
/*
Set the data characters that are encoded into UPC-A barcode symbology.
Valid data set of UPC-A consists of,
Numeric Digits: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
UPC-A barcode encodes a fixed data length of 11 data digits plus 1 check digit
*/
barcode.CodeText = "12345678901";
/*
Enbable the checksum option for UPC-A
so that the barcode generator computes check digit to UPC-A automatically
*/
barcode.EnableChecksum = true;
//Set the barcode graphic measurements as Pixel for UPC-A
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Assign values of bar width and bar height to UPC-A barcode
barcode.X = 1;
barcode.Y = 75;
// Set barcode symbol width & height of generated UPC-A barcode
barcode .BarCodeWidth =140;
barcode .BarCodeHeight =120;
//Set the margins around the UPC-A symbol
barcode.LeftMargin = 10;
barcode.RightMargin = 10;
barcode.TopMargin = 10;
barcode.BottomMargin = 10;
/*
Assign a value to resolution for UPC-A image that is drawn to,
and change the orientation of UPC-A barcode image
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
/*
Display the characters encoded into the UPC-A symbology and
set the font style of the characters encoded into EAN128
*/
barcode.DisplayCodeText = true;
barcode.CodeTextFont = new System.Drawing.Font("Arial", 11f, System.Drawing.FontStyle.Regular);
/*
Set UPC-A barcode drawing image format to PNG in C# and
generate UPC-A image in C#
*/
barcode.Format = System.Drawing .Imaging .ImageFormat.Png;
barcode.drawBarcode("C://barcode-upca-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Generate other Linear & 2D Barcode Images using C#.NET PDF417 Bacoding Library
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.