C# .NET EAN-13 Barcode Generator Library
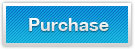
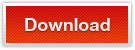
C# .NET EAN-13 Barcode Generator Library encodes, generates EAN13 barcode labels in Visual Studio 2005/2008/2010 using C# class codes.
Overview - C# .NET EAN-13 Barcode Generator Control
EAN-13, a numeric-only barcode type, is used for consumer products internationally. This linear barcode can encode 13-digit data, including a check digit. This .NET EAN-13 barcode creating sdk can generate industry-standard EAN-13 barcode images using C# class codes. It support EAN-13 barcode generation in .NET 2.0, 3.0, 3.5, 4.o and above framework application.
Popular barcoding solutions for generating & printing EAN-13 barcode image in .NET application using C# class code:
Features - EAN-13 Barcoding Component for C#.NET Class Application
Technologies of EAN-13 C# .NET Barcode Generator Library
- Mature EAN-13 barcode generating library for EAN-13 barcode generation using C# code
- Entirely built in .NET 2.0 and supports .NET 2.0, 3.0, 3.5, 4.0 framework applications
- Able to generate & create EAN-13 barcode images in C# .NET classes and Console applications
- Generate & output EAN-13 barcode images in in ASP.NET web applicaton, Windows Forms project, SQL Server Reporting Services (SSRS), RDLC and Crystal Reports
Barcode Imaging of EAN-13 C# .NET Barcode Generator Library
Full functional features of C# .NET EAN-13 Barcode Generator Component make the operation of barcode easy and simple.
- Using this EAN-13 C#.NET barcoding library, generated EAN-13 barcode images can be saved to gif, jpeg, png, bmp, and tiff and memory files
- This EAN-13 C#.NET barcoding dll can generate EAN-13 barcode images with four possible generating directions
- This barcode creating library supports hiding or displaying human-readable EAN-13 barcode images in C#.NET class project
- Generate & adjust EAN-13 barcode images size, like bar width, margins & barcode width & height using C#.NET class code
Generate EAN-13 Barcode Images Using Free C# Class Code
/*
Create a new linear barcode object in C#.NET,
and change the creating symbology to EAN13 barcode type
*/
BarCode barcode = new BarCode();
barcode.SymbologyType = SymbologyType.EAN13;
/*
Assign 12 data digits that are encoded into EAN13 barcode symbology.
Encodable character set of EAN13 is as follow,
Numeric characters: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9
EAN13 symbology encodes a fixed data length of 13 digits inclusive of the check digit
*/
barcode.CodeText = "123456789012";
/*
Enable the checksum option for EAN13 barcode symbology
so that the barcode library computes the check digit automatically
*/
barcode.EnableChecksum = true;
//Select the graphic measurements as Pixel to EAN13 barcode
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Set the values of bar width and bar height to EAN13 barcode
barcode.X = 1;
barcode.Y = 75;
//Set the margin distance around the EAN13 barcode symbol
barcode.LeftMargin = 10;
barcode.RightMargin = 10;
barcode.TopMargin = 10;
barcode.BottomMargin = 10;
// Adjust generate EAN-13 barcode symbol width & height
barcode.BarCodeWidth = 150;
barcode.BarCodeHeight = 120;
/*
Set the resolution of EAN13 barcode image that is drawn to,
and change the EAN13 barcode image orientation
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
/*
Display the characters encoded into the EAN13 barcode symbology
Set the font style of characters encoded into the EAN13 barcode
*/
barcode.DisplayCodeText = true;
barcode.CodeTextFont = new System.Drawing.Font("Arial", 11f, System.Drawing.FontStyle.Regular);
/*
Set EAN13 barcode drawing image format to PNG in C#.NET
Generate EAN13 barcode image in C#
*/
barcode.Format = System.Drawing. Imaging.ImageFormat.Png;
barcode.drawBarcode("C://barcode-ean13-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Generate other 1D & 2D Barcode Images Using EAN-13 C#.NET Barcoding Library
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.