VB.NET Barcode Generation Tutorial
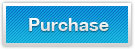
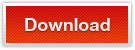
KeepDynamic offers the most affordable .NET barcode generator licenses for adding barcode creation features into VB.NET projects. Please visit the online purchase page. This tutorial illustrates how to generate barcodes in VB.NET projects with Visual Basic .NET code using KeepDynamic's Barcode SDK. If you are a C#.NET developer, please go to the
C# code for generating barcode. KeepDynamic aims to help you easily and simply create both linear (1D) and Matrix (2D) barcodes in your Microsoft .NET applications. You can use the
Barcode SDK for .NET Windows Forms to add barcode features into .NET Windows applications and use
ASP.NET Barcode Generator SDK to generate barcodes in ASP.NET web applications.
More than 20 linear and 2D barcodes are able to be generated using our barcode component in VB.NET for .NET projects, such as:
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- Numeric Barcodes: Identcode, Leitcode, Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.
Features of VB.NET Barcode Generator Component
Our Barcode Generator for VB.NET is completely developed in .NET 2.0, and compatible with the .NET 2.0 and greater development environments. You can easily integrate it into your VB.NET applications. Both linear and matrix barcode are able to be immediately generated in your VB.NET Classes and console applications. In VB.NET, ASP.NET web projects, and VB.NET Windows Forms software, barcode images can be easily created. This VB.NET Barcode Generator also supports barcode generating in Reporting Service & Crystal Reports document.
Barcode Images Generated by VB.NET Barcode Generating SDK
This Barcode Generator is capable of generating barcodes using Visual Basic .NET in image formats such as JPEG, GIF, TIFF, BMP, and PNG. Properties of barcode images, such as the barcode size, are able to be modulated based on users' requirements and the image orientation may be set to 0, 90, 180, or 270 degrees. With the thermal printer support, high quality barcode images are able to be printed even on low resolution printers as well as on high resolution printers. White bar increase option widens the white space between the bars for improving the readability.
Customizing Barcode Image Settings Using Visual Basic .NET
Barcode generator provides diversified options for easily customizing the barcodes in VB.NET. Text Options enables or disables the human-readable text for linear barcodes in VB. NET. Checksum Options may enable the human-readable checksum character appeared or not, however, the checksum character is actually encoded into the linear Barcode. Graphic Configuration Options configure the colors of the foreground and background. Tilde Functions allow the functions, such as returns, tabs and other ones, to be encoded easily. Bearer bar for ITF14 and linear barcode are easy to be generated.
How to Install Barcode Creating Control to VB.NET projects
Generating Barcodes Using .NET Windows Form Barcode Generator Control
To create barcodes in VB.NET Windows Forms applications, do the following procedures:
- Download the Barcode SDK for .NET package and unzip the suite
- Open the VB.NET Windows Application that you are going to create barcodes or create a new one
- Add KeepDynamic.BarCode.Windows.dll to your VB.NET project reference
- Add KeepDynamic.BarCode.Windows.dll to windows project toolbox
- Drag one of the BarCodeControl, DataMatrixControl, PDF417Control, QRCodeControl item to your windows forms
Creating Barcodes for ASP.NET applications using ASP.NET barcode generator
To create barcodes in VB.NET ASP.NET Web Forms applications, do the following procedures:
- Download the .NET barcode control suite and unzip the package
- Open the ASP.NET Web project or create a new ASP.NET web application in VB.NET
- Add KeepDynamic.BarCode.AspNet.dll to your ASP.NET project reference
- Add KeepDynamic.BarCode.AspNet.dll to ASP.NET Web project toolbox
- Copy barcode folder to your asp.net project
- Drag the one of the BarCodeControl, DataMatrixControl, PDF417Control, QRCodeControl item to your ASP.NET Web form pages
How to Generate Linear (1D) Barcodes in VB.NET Class?
The following VB.NET code illustrates how to generate linear barcodes in VB.NET class:
' Create & Generate linear barcode object in VB.NET
Dim code128 As New BarCode()
' Set barcode type to Code-128 in VB.NET
code128.SymbologyType = SymbologyType.Code128
' Set barcode encodable value in VB.NET
code128.CodeText = "Code128"
' Adjust Code 128 barcode image size
code128.BarCodeWidth = 150
code128.BarCodeHeight = 90
' Set barcode drawing image format to PNG in VB.NET
code128.Format = System.Drawing.Imaging.ImageFormat.Png
' Output generated barcode image to the png image file in VB.NET
code128.drawBarcode("C://barcode-code128-vbnet.png")
How to Generate Data Matrix Barcodes in VB.NET Class?
The following VB.NET code illustrates how to generate Data Matrix barcodes in VB.NET class:
' Generate & print Data Matrix barcode object in VB.NET
Dim barcode As New DataMatrix()
' Set Data Matrix barcode value in VB.NET
barcode.CodeText = "Data Matrix in VB.NET"
' customize data matrix barcode image size settings
barcode.X = 4
barcode.TopMargin = 4
barcode.RightMargin = 4
barcode.LeftMargin = 4
barcode.BottomMargin = 4
' Set Data Matrix barcode data mode
barcode.DataMode = DataMatrixDataMode.ASCII
' Save created barcode image to the png image in VB.NET
barcode.drawBarcode("C://barcode-datamatrix-vbnet.png")
How to Generate PDF-417 Barcodes in VB.NET Class?
The following VB.NET code illustrates how to generate PDF-417 barcodes in VB.NET class:
' Create PDF417 barcode object in using VB.NET
Dim barcode As New PDF417()
' Set PDF-417 barcode value in using VB.NET
barcode.CodeText = "PDF417 using VB.NET"
' set unique PDF 417 barcode image settings
barcode.ColumnCount = 3
barcode.RowCount = 10
barcode.X = 3
barcode.XtoYRatio = 0.3333333F
' Set PDF-417 drawing image format to PNG in using VB.NET
barcode.Format = System.Drawing.Imaging.ImageFormat.Png
' Generate PDF-417 barcode & encode to the png image using VB.NET
barcode.drawBarcode("C://barcode-pdf417-vbnet.png")
How to Generate QR-Code Barcodes in VB.NET Class?
The following VB.NET code illustrates how to generate QR-Code barcodes in VB.NET class:
' Used to generate QR Code barcode image
Dim barcode As New QRCode()
' Used to encode characters to QR Code barcode
barcode.CodeText = "QRCode in VB.NET"
' Set QR Code module size
barcode.X = 4
' Set QR Code quiet zone
barcode.BottomMargin = 16
barcode.LeftMargin = 16
barcode.RightMargin = 16
barcode.TopMargin = 16
'Set QR Code error correction level
barcode.ECL = QRCodeECL.L
' Output generated qr code to png image file
barcode.drawBarcode("C://barcode-qrcode-vbnet.png")