GS1-128 Barcode Generator Library for C#.NET Application
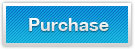
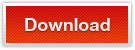
GS1-128 Barcode Generator Library for C#.NET Application is a mature & advanced barcoding solution for creating & generating EAN128, UCC128, or GS1-128 barcodes in .NET application using C# class code.
Overview - C#.NET GS1-128/EAN-128 Barcode Generator DLL
Derived from Code 128 barcode image, GS1-128 barcode symbology is used to encode shipping/product information. This GS1-128/EAN-128 barcoding library can generate & create GS1-128/EAN-128 barcode images in .NET framework projects using C# class codes.
Related barcoding solutions for generating EAN-128 image in .NET application using C# class codes:
Features - C#.NET GS1-128/EAN-128 Barcode Generator DLL
Technologies of GS1-128/UCC/EAN-128 C# .NET Generator Library
- Built in managed C# code and support GS1-128/UCC/EAN-128 barcode generation in C#.NET application
- Developed in .NET 2.0 and support EAN-128 barcode generation in .NET 2.0, 3.0, 3.5, 4.0
- Able to create GS1-128 barcodes in ASP.NET web projects, Microsoft Windows Forms, SQL Server Reporting Services (SSRS), Local Reports RDLC and Crystal Reports
Barcoding Customization of GS1-128/UCC/EAN-128 C# .NET Generator Library
- Generated GS1-128/UCC/EAN-128 can be saved to gif, jpeg, png, bmp, and tiff image files and byte stream object using C# code
- Generate GS1-128/UCC/EAN-128 barcode images in 0, 90, 180, or 270 degrees in C#.NET class project
- Create & print GS1-128/UCC/EAN-128 barcode images with different resolutions using C# code
- Adjust generated GS1-128/UCC/EAN-128 image size using C# code, like bar width, barcode size
- Hide or display human-readable text of generated GS1-128/UCC/EAN-128 barcode images using C# code
- Offer Titldprocess function to encode special characters in C#.NET class code
C# Code for Creating GS1-128(UCC/EAN-128) Barcode Images in .NET Applications
/*
Create a new linear barcode object in C#.NET
and select the barcode symbology as EAN128 from the barcode type list
*/
BarCode barcode = new BarCode();
barcode.SymbologyType = SymbologyType.EAN128;
/*
Set the data characters that are encoded into EAN128 barcode symbology.
Encodable data set of EAN128 contains,
All 128 ASCII characters.
EAN128 symbol encodes variable length of data characters
The encodable data should be put in the format (AI)+data
*/
barcode.CodeText = "(12)3515558";
// Activate ProcessTilde funtion, after which you can encode data in the format of ~ai2123515558
barcode.ProcessTilde = true;
/*
Enbable the checksum option for EAN128
so that the barcode generator computes check digit to EAN128 automatically
*/
barcode.EnableChecksum = true;
//Set the barcode graphic measurements as Pixel for EAN128
barcode.GraphicsUnit = KeepDynamic.Barcode.Generator.GraphicsUnit.Pixel;
//Assign values of bar width and bar height to EAN128 barcode
barcode.X = 1;
barcode.Y = 75;
//Set the margins around the EAN128 symbol
barcode.LeftMargin = 10;
barcode.RightMargin = 10;
barcode.TopMargin = 10;
barcode.BottomMargin = 10;
// Set EAN-128 barcode image size
barcode.BarCodeHeight = 120;
barcode.BarCodeWidth = 160;
/*
Assign a value to resolution for EAN128 image that is drawn to,
and change the orientation of EAN128 barcode image
*/
barcode.Resolution = 72;
barcode.Rotate = Rotate.Rotate0;
/*
Display the characters encoded into the EAN128 symbology and
set the font style of the characters encoded into EAN128
*/
barcode.DisplayCodeText = true;
barcode.CodeTextFont = new System.Drawing.Font("Arial", 11f, System.Drawing.FontStyle.Regular);
/*
Set EAN128 barcode drawing image format to PNG in C# and
generate EAN128 image in C#
*/
barcode.Format = System.Drawing.Imaging.ImageFormat.Png;
barcode.drawBarcode("C://barcode-ean128-csharp.png");
// other barcode outputting ways:
// output generated barcode to byte array
byte[] barcodeInBytes = barcode.drawBarcodeAsBytes();
// output generated barcode to Graphics object
Graphics graphics = ...;
barcode.drawBarcode(graphics);
// output generated barcode to Bitmap object
Bitmap barcodeInBitmap = barcode.drawBarcode();
// draw created barcode to HttpResponse object
HttpResponse response = ...;
barcode.drawBarcode(response);
// save generated barcode images to Stream object
Stream stream = ...;
barcode.drawBarcode(stream);
Supported Barcodes by C#.NET GS1-128(UCC/EAN-128) Barcoding Library
- 2D Barcodes: Data Matrix, PDF-417 and QR-Code.
- Alphanumeric Barcodes: Code-39, Code-93, Code-128 and GS1-128/UCC/EAN-128.
- UPC / EAN Barcodes: EAN-8, EAN-13, ISBN, ISSN, UPC-A and UPC-E.
- Numeric Barcodes: Identcode, Leitcode,Codabar and Code-11.
- Code 2 of 5 based Barcodes: Code 2 of 5, Interleaved 2 of 5 and ITF-14.
- Postal Barcodes: POSTNET, PLANET, USPS Intelligent Mail Barcode (OneCode), RM4SCC and MSI Plessey.